Introduction :
The DHT11 is a basic, low-cost digital temperature and humidity sensor. It uses a capacitive humidity sensor and a thermistor to measure the surrounding air, and it outputs a digital signal on the data pin (no analog input pins needed)
Key Features
- Temperature Range: 0 to 50°C (±2°C accuracy)
- Humidity Range: 20% to 90% RH (±5% accuracy)
- Operating Voltage: 3.5V to 5.5V
- Max Current: 2.5mA (during conversion)
- Output: Digital signal via single-bus interface
Pin Configuration
- VCC: Power supply (3.5V to 5.5V)
- Data: Serial data output (connect to a digital pin on the microcontroller)
- GND: Ground
Connection:
- VCC to 5V (Arduino)
- GND to GND (Arduino)
- Data to a digital pin (e.g., pin 4) on the Arduino
Code :
#include <DHT.h>
#define DHTPIN 4 // Pin connected to the Data pin of DHT11
#define DHTTYPE DHT11 // DHT 11 sensor type
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 baud
dht.begin(); // Initialize the DHT sensor
}
void loop() {
delay(2000); // Wait a few seconds between measurements
float humidity = dht.readHumidity(); // Read humidity
float temperatureC = dht.readTemperature(); // Read temperature as Celsius
float temperatureF = dht.readTemperature(true); // Read temperature as Fahrenheit
// Check if any reads failed and exit early (to try again).
if (isnan(humidity) || isnan(temperatureC) || isnan(temperatureF)) {
Serial.println(“Failed to read from DHT sensor!”);
return;
}
Serial.print(“Humidity: “);
Serial.print(humidity);
Serial.print(” %\t”);
Serial.print(“Temperature: “);
Serial.print(temperatureC);
Serial.print(” *C “);
Serial.print(temperatureF);
Serial.println(” *F”);
}
https://github.com/makertribe/IoT-Codes/blob/8827f3514542eaead0f456b647918363b2247cc6/temperature%20sensor%20code
Output:
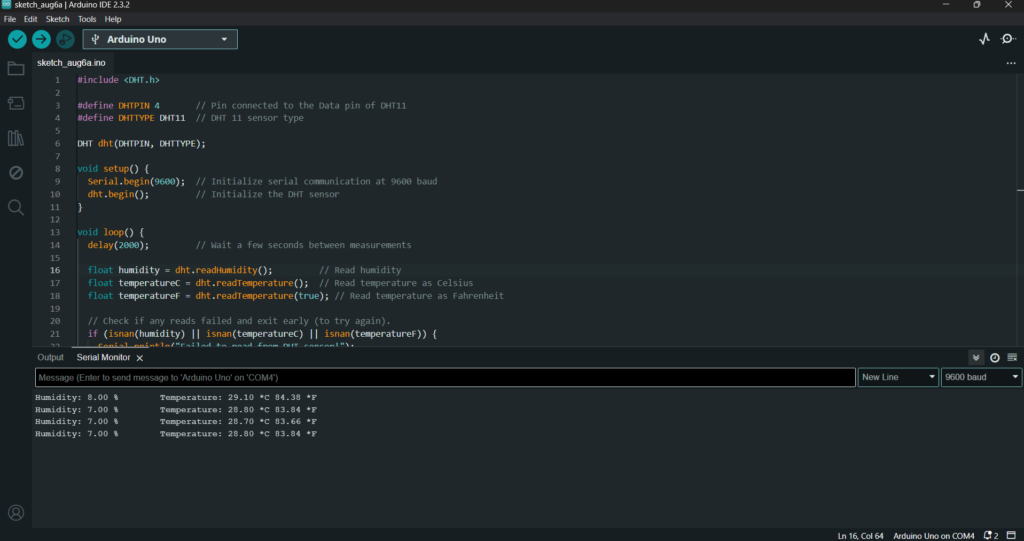
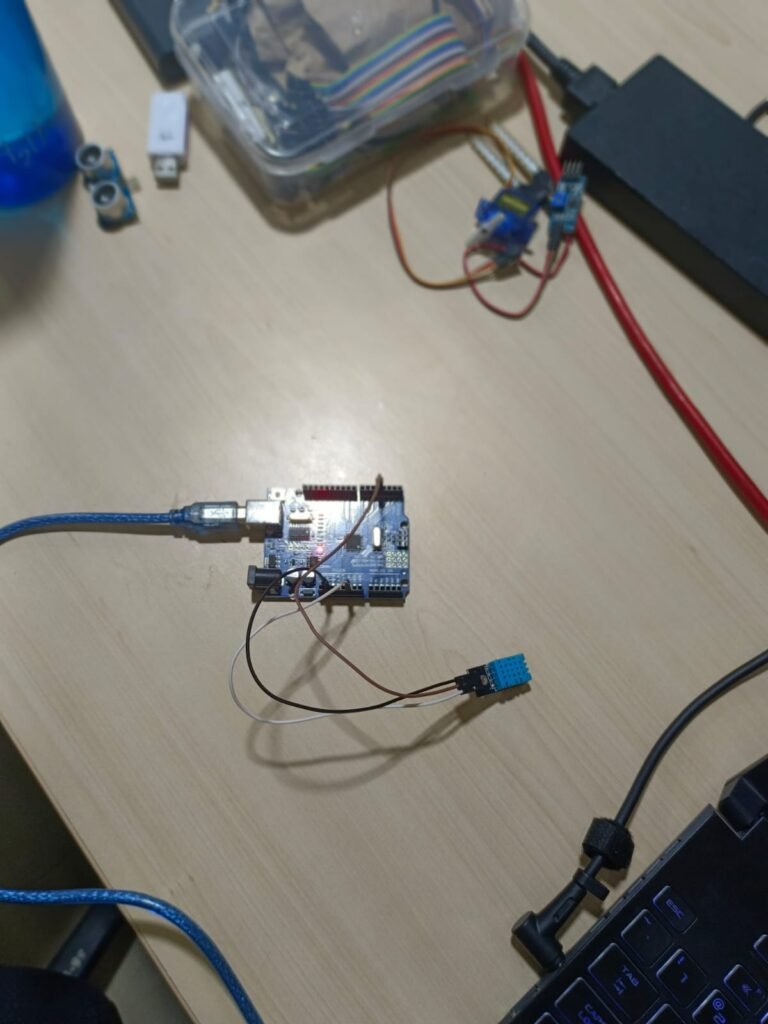